Golang中的虚拟机实现一步步构建你自己的解释器
Golang中的虚拟机实现:一步步构建你自己的解释器
在本文中,我们将介绍如何使用Golang实现一个简单的解释器,以便了解虚拟机背后的工作原理。我们将使用Golang作为我们的开发语言,因为它是一种现代且易于使用的语言,具有出色的并发性。本文中将会覆盖的主题包括:
- 解释器的概念介绍
- 词法分析器
- 语法分析器
- 虚拟机的实现
什么是解释器?
解释器是一种可以读取和执行源代码的程序。与编译器不同,解释器在执行程序时不需要将其编译成机器码。相反,解释器会读取源代码并直接执行它。解释器的优点是它可以逐行执行代码,这使得调试更加容易。但是,解释器的缺点是它通常比编译器慢。
词法分析器
解释器通常从源代码中读取字符并将其转换为有意义的标记(token)。词法分析器就负责这个任务。在我们的解释器中,我们将使用正则表达式来匹配标记。
下面是我们解释器的标记:
- 整数(int)
- 加号(+)
- 减号(-)
- 左括号(()
- 右括号())
接下来,我们将编写正则表达式来匹配这些标记:
`go
const (
INT = "INT"
PLUS = "PLUS"
MINUS = "MINUS"
LPAREN = "LPAREN"
RPAREN = "RPAREN"
)
type Token struct {
Type string
Value string
}
type Lexer struct {
input string
position int
currentToken Token
}
func NewLexer(input string) *Lexer {
l := &Lexer{input: input}
l.position = -1
return l
}
func (l *Lexer) NextToken() Token {
l.skipWhiteSpace()
if l.peek() == '+' {
l.advance()
return Token{Type: PLUS, Value: "+"}
} else if l.peek() == '-' {
l.advance()
return Token{Type: MINUS, Value: "-"}
} else if l.peek() == '(' {
l.advance()
return Token{Type: LPAREN, Value: "("}
} else if l.peek() == ')' {
l.advance()
return Token{Type: RPAREN, Value: ")"}
} else if isDigit(l.peek()) {
return Token{Type: INT, Value: l.readNumber()}
}
return Token{}
}
func (l *Lexer) readNumber() string {
result := ""
for isDigit(l.peek()) {
result += string(l.peek())
l.advance()
}
return result
}
func (l *Lexer) skipWhiteSpace() {
for l.peek() == ' ' || l.peek() == '\t' || l.peek() == '\n' || l.peek() == '\r' {
l.advance()
}
}
func (l *Lexer) advance() {
l.position++
if l.position < len(l.input) {
l.currentToken = Token{Type: "", Value: string(l.input)}
} else {
l.currentToken = Token{}
}
}
func (l *Lexer) peek() byte {
if l.position+1 < len(l.input) {
return l.input
} else {
return byte(0)
}
}
func isDigit(char byte) bool {
return char >= '0' && char <= '9'
}
在上述代码中,我们定义了一个Lexer结构体,它维护解释器的输入,当前标记及其位置。我们使用NextToken方法从输入中获取下一个标记。使用isDigit函数来确定标记是否为整数,并使用readNumber来读取整数的数字。skipWhiteSpace用于跳过源代码中的空格。语法分析器在语法分析器中,我们将根据词法分析器返回的标记构建一个代表程序结构的树状结构。在我们的解释器中,我们将使用递归下降的方法来构建树。下面是我们解释器的文法:
expression ::= term ((PLUS|MINUS) term)*
term ::= factor ((MUL|DIV) factor)*
factor ::= INT | LPAREN expression RPAREN
我们将在语法分析器中实现这个文法。我们使用Term方法来处理级别较低的操作符,例如乘法和除法,并使用Expression方法处理加法和减法。`gotype Parser struct { lexer *Lexer currentToken Token}func NewParser(lexer *Lexer) *Parser { p := &Parser{lexer: lexer} p.currentToken = lexer.NextToken() return p}func (p *Parser) Parse() Node { return p.Expression()}func (p *Parser) Expression() Node { node := p.Term() for p.currentToken.Type == PLUS || p.currentToken.Type == MINUS { token := p.currentToken if token.Type == PLUS { p.eat(PLUS) } else if token.Type == MINUS { p.eat(MINUS) } node = &BinaryOpNode{Left: node, Op: token, Right: p.Term()} } return node}func (p *Parser) Term() Node { node := p.Factor() for p.currentToken.Type == MUL || p.currentToken.Type == DIV { token := p.currentToken if token.Type == MUL { p.eat(MUL) } else if token.Type == DIV { p.eat(DIV) } node = &BinaryOpNode{Left: node, Op: token, Right: p.Factor()} } return node}func (p *Parser) Factor() Node { token := p.currentToken if token.Type == INT { p.eat(INT) return &NumNode{Token: token} } else if token.Type == LPAREN { p.eat(LPAREN) node := p.Expression() p.eat(RPAREN) return node } else { return nil }}func (p *Parser) eat(tokenType string) { if p.currentToken.Type == tokenType { p.currentToken = p.lexer.NextToken() } else { panic("unexpected token") }}
在Parser结构体中,我们使用currentToken变量维护当前标记,并使用eat方法来从输入中读取下一个标记。
虚拟机的实现
我们的虚拟机将会解释抽象语法树,执行树中的操作并返回结果。我们将使用接口定义节点的行为,并使用具体类型实现这些接口。在我们的解释器中,我们的抽象语法树将包括以下节点:
- NumNode:表示整数值的节点
- BinaryOpNode:表示二元操作符的节点
下面是我们的节点接口和具体实现:
`go
type Node interface {
Eval() int
}
type NumNode struct {
Token Token
}
func (n *NumNode) Eval() int {
i, _ := strconv.Atoi(n.Token.Value)
return i
}
type BinaryOpNode struct {
Left Node
Op Token
Right Node
}
func (n *BinaryOpNode) Eval() int {
if n.Op.Type == PLUS {
return n.Left.Eval() + n.Right.Eval()
} else if n.Op.Type == MINUS {
return n.Left.Eval() - n.Right.Eval()
} else if n.Op.Type == MUL {
return n.Left.Eval() * n.Right.Eval()
} else if n.Op.Type == DIV {
return n.Left.Eval() / n.Right.Eval()
} else {
return 0
}
}
在上述代码中,我们实现了Node接口中的Eval方法。对于NumNode,Eval将返回节点代表的整数值。对于BinaryOpNode,Eval将递归计算左右子节点并执行相应的操作符。最后,我们将实现我们的虚拟机。这个虚拟机将会使用语法分析器构建的抽象语法树并执行它。`gotype Interpreter struct { parser *Parser}func NewInterpreter(parser *Parser) *Interpreter { return &Interpreter{parser: parser}}func (i *Interpreter) Interpret() int { tree := i.parser.Parse() return tree.Eval()}
在上述代码中,我们实现了一个Interpreter结构体并使用parser构建了抽象语法树。最后,我们使用Interpret方法执行抽象语法树并返回结果。
总结
在本文中,我们使用Golang实现了一个简单的解释器,并了解了其背后的工作原理。我们涵盖了解释器的概念,词法分析器,语法分析器和虚拟机的实现。我们还介绍了在语法分析器中使用递归下降方法来构建抽象语法树,并介绍了如何在虚拟机中执行树并返回结果。

猜你喜欢LIKE
相关推荐HOT
更多>>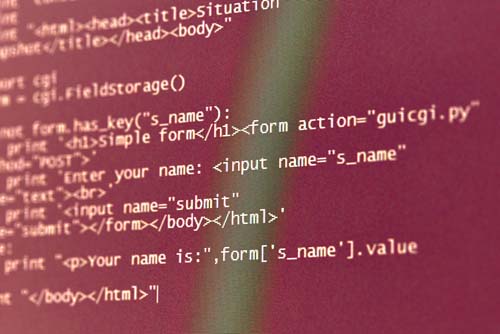
云计算建立了新的计算和存储模式
随着云计算技术的发展,越来越多的企业和用户开始将自己的计算和存储需求转移到云端。这种新的计算和存储模式不仅能够为用户提供更高效、更稳定...详情>>
2023-12-21 22:32:46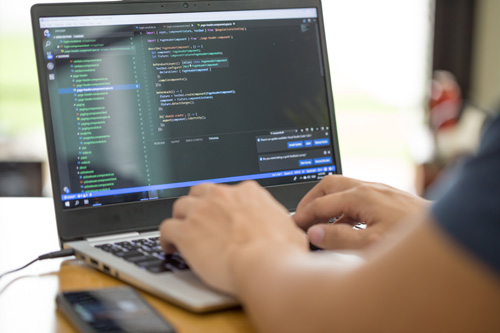
如何使用Golang实现微服务架构下的分布式事务?
如何使用Golang实现微服务架构下的分布式事务?在现代化的应用程序中,微服务架构的使用已经成为了主流。然而,随着应用程序的复杂性增加以及系...详情>>
2023-12-21 18:56:46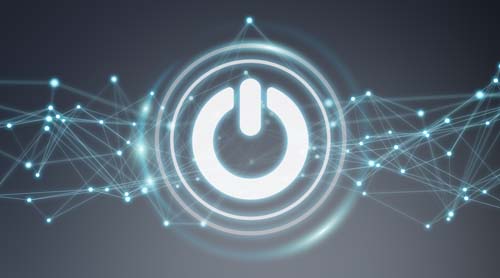
如何在Goland中优雅地处理Go项目的依赖管理?
如何在Goland中优雅地处理Go项目的依赖管理?在Go项目中,依赖管理是一个非常重要的环节。优秀的依赖管理可以使我们的项目更加健壮和可靠,但是...详情>>
2023-12-21 04:32:45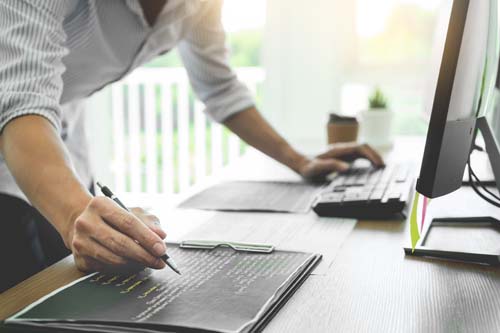
Goland集成Jenkins实现自动化部署的技巧
Goland集成Jenkins实现自动化部署的技巧在现代软件开发中,自动化部署已经成为了不可或缺的一部分。自动化部署可以减少人工干预,减少出错的概...详情>>
2023-12-20 23:44:45热门推荐
Linux集群管理实现高可用性
沸云计算建立了新的计算和存储模式
热小心翼翼:如何保护你的个人数据
热什么是端口扫描?如何检测并防范
新如何使用Golang实现微服务架构下的分布式事务?
Golang实战通过Docker部署Web应用程序
Golang与MongoDB使用mgo进行数据操作
Golang中的虚拟机实现一步步构建你自己的解释器
Golang高并发编程实战如何避免死锁和数据竞态?
Go并发编程指南使用Goland构建高性能并发程序
Goland中的代码导航快速定位并编辑您需要的代码
使用Goland的远程调试功能进行强大而高效的调试
编写灵活的Go语言代码Goland的实用技巧和技巧
Goland项目管理技巧,让你的团队协作更加无缝!
技术干货
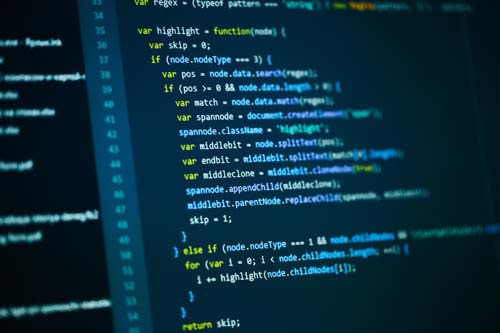
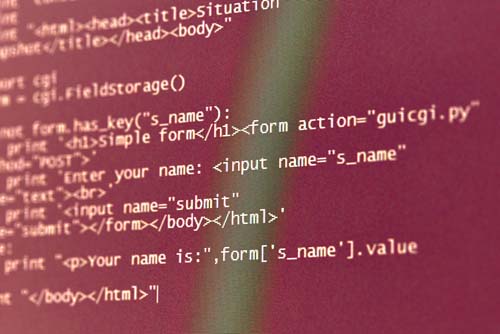
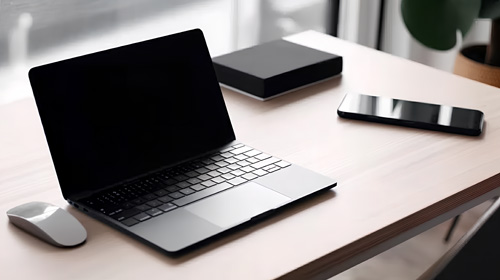
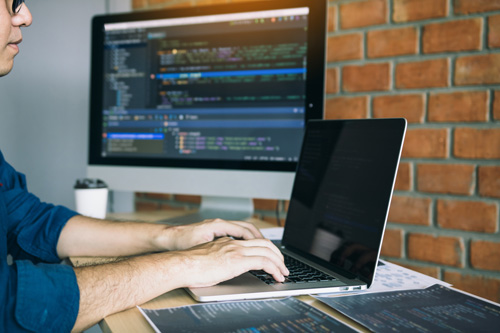
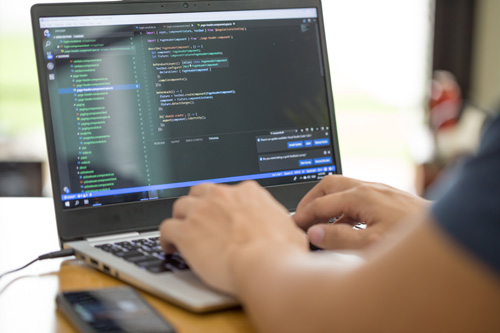
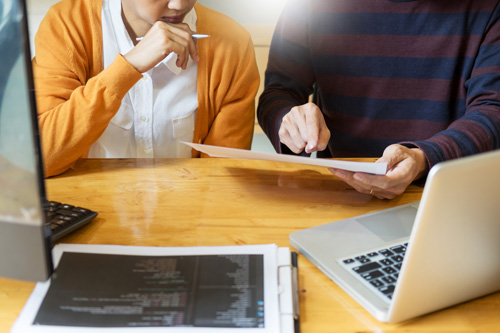
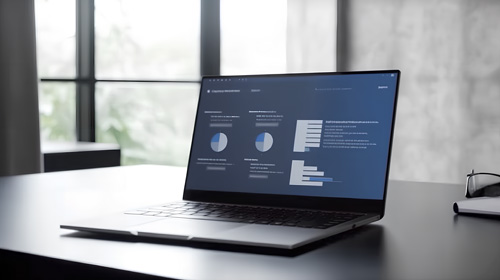